Deploy Frontend Application to AWS S3 using Circle CI
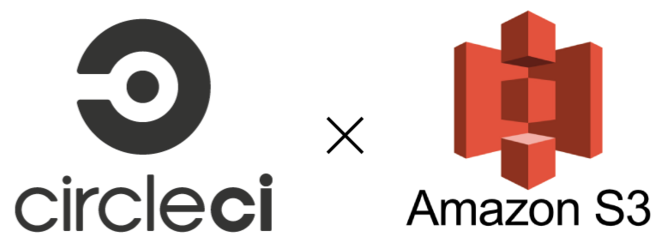
If you are hosting your frontend application on AWS S3 using static hosting, automating the deployment process is something you might be interested in. If so, keep reading.
What is Circle CI?
Circle CI is a continuous integration tool that can help you test, build and deploy your code as soon as you push new commit or make a new release. If you don’t have a CircleCI account already, go ahead and sign up using your GitHub/BitBucket account.
Create AWS Access Key/Secret
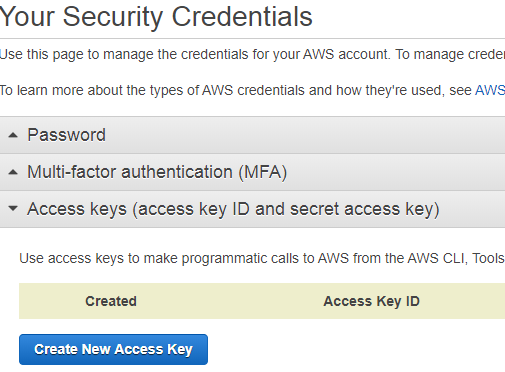
Log in to your AWS account and navigate to My Security Credentials page. Under “Access keys (access key ID and secret access key)” tab, click “Create New Access Key”, once created download the key file and keep it safe we will be using it later.
Creating Circle CI config.yml
In your repository’s root, create a folder named .circleci
and add a file config.yml
(so that the file path be in .circleci/config.yml). Now let’s start writing our configuration.
Version
The first line should mention the version of CircleCI your config is going to target. Since we are going to use Orbs, we will pick the version 2.1.
version: 2.1
Orbs
Next, we will define the orbs that we will be using in our project. We are going to use aws-cli
orb to upload files to S3.
orbs:
aws-cli: circleci/aws-cli@0.1.18
Create our Jobs
Jobs are just a group of steps to be performed. We will be creating 2 jobs namely:
- build_project – responsible to build the project.
- deploy_project – responsible to upload the built files to S3.
version: 2.1
jobs:
build_project:
working_directory: ~/circle-ci-s3-deploy
docker:
- image: node:10.15.3
steps:
- checkout
- run:
name: Installing dependencies
command: yarn
- run:
name: Building project
command: yarn build
- persist_to_workspace:
root: .
paths:
- .
deploy_project:
executor: aws-cli/default
steps:
- attach_workspace:
at: .
- aws-cli/setup:
profile-name: default
- run:
name: Upload file to S3
command: aws s3 sync ./dist/ s3://circle-ci-s3-deploy --delete
There is nothing fancy in build_project
job. We are just cloning our repository, installing our dependencies and then building the project. One thing to note is how we persist the working directory to the workspace so that the built files are available to the next job.
In deploy_project
job, we specify that this job is to be executed by aws-cli orb using the executor
property. We then attach the persisted workspace so that we get all the built files from the previous jobs and then use a command aws-cli/setup
provided by the aws-cli orb to install aws-cli. Finally, we will run the plain AWS command to sync our built files to the bucket.
Defining the Workflow
Workflows are used to schedule and sequence jobs.
workflows:
version: 2
build:
jobs:
- build_project:
filters:
branches:
ignore: /.*/
tags:
only: /.*/
- deploy_project:
requires:
- build_project
context: aws-context
filters:
branches:
ignore: /.*/
tags:
only: /.*/
The important part here is the context
provided to deploy_project
job. Contexts are CircleCI’s way to inject environment variables into the Job. We will be creating contexts in the next step to inject AWS Key/Secret that we created earlier.
We need the build_project job to run first, then followed by the deploy_project job. We can configure that by saying deploy_project
job requires
the build_project
job.
We use the filters
property to make our job run only when a new release is tagged.
Creating Circle CI Context
We can create a context from the CircleCI organization settings page. Make sure you name the context same as what we declared in config.yml
i.e, aws-context
Once the context is created, you can add environment variables to the context. The aws-cli
orb requires 3 environment variables namely:
- AWS_ACCESS_KEY_ID
- AWS_SECRET_ACCESS_KEY
- AWS_DEFAULT_REGION
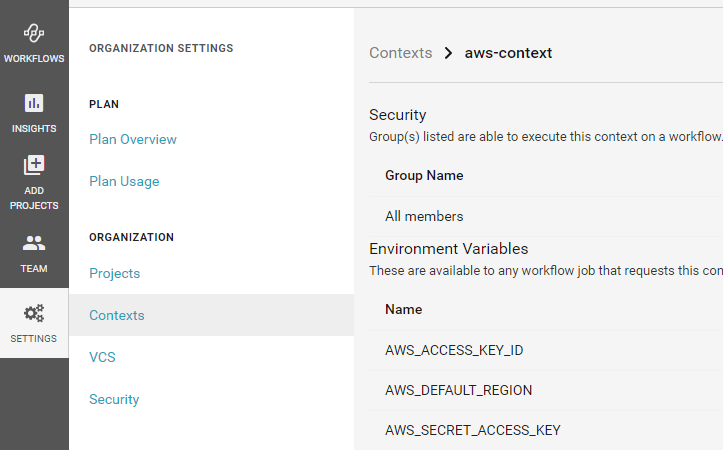
You can give the give AWS access key and secret that we created earlier for AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY respectively. AWS_DEFAULT_REGION is the AWS region code like us-east-1, choose this based on your preference.
That’s all folk. Go ahead and tag a new release on Git/Bitbucket and see your code getting deployed automatically.
You can find the full code here
P.S This is just a simple use case. We can use Circle CI to build docker images, upload it to Docker hub, deploy the Docker image to the Cloud and a lot of other cool things, so ahead and start experimenting.